PySimpleGUI - Graph 图形元素
Graph 元素类似于 Canvas,但非常强大。 您可以定义自己的坐标系,使用您自己定义的单位,然后将它们显示在以像素为单位定义的区域中。
您应该为 Graph 对象提供以下值 −
画布大小(以像素为单位)
您坐标系的左下角 (x,y) 坐标
坐标系的右上角 (x,y) 坐标
Graph 创建图形,并通过调用以下方法获取图形ID,类似于Tkinter Canvas −
draw_arc(self, top_left, bottom_right, extent, start_angle, style=None, arc_color='black', line_width=1, fill_color=None) draw_circle(self, center_location, radius, fill_color=None, line_color='black', line_width=1) draw_image(self, filename=None, data=None, location=(None, None)) draw_line(self, point_from, point_to, color='black', width=1) draw_lines(self, points, color='black', width=1) draw_oval(self, top_left, bottom_right, fill_color=None, line_color=None, line_width=1) draw_point(self, point, size=2, color='black') draw_polygon(self, points, fill_color=None, line_color=None, line_width=None) draw_rectangle(self, top_left, bottom_right, fill_color=None, line_color=None, line_width=None) draw_text(self, text, location, color='black', font=None, angle=0, text_location='center')
除了上述绘制方法外,Graph 类还定义了 move_figure() 方法,通过该方法,通过提供相对于其先前坐标的新坐标,将由其 ID 标识的图像移动到其新位置 .
move_figure(self, figure, x_direction, y_direction)
如果将 drag_submits 属性设置为 True,则可以捕获图形区域内的鼠标事件。 当你点击图形区域的任意位置时,产生的事件是:Graph_key + '+UP'。
在下面的示例中,我们在图形元素的中心绘制一个小圆圈。 在图形对象下方,有左右移动圆圈的按钮。 单击时,将调用 mov_figure() 方法。
import PySimpleGUI as psg graph=psg.Graph(canvas_size=(700,300), graph_bottom_left=(0, 0), graph_top_right=(700,300), background_color='red', enable_events=True, drag_submits=True, key='graph') layout = [[graph], [psg.Button('LEFT'), psg.Button('RIGHT'), psg.Button('UP'), psg.Button('DOWN')]] window = psg.Window('Graph test', layout, finalize=True) x1,y1 = 350,150 circle = graph.draw_circle((x1,y1), 10, fill_color='black', line_color='white') rectangle = graph.draw_rectangle((50,50), (650,250), line_color='purple') while True: event, values = window.read() if event == psg.WIN_CLOSED: break if event == 'RIGHT': graph.MoveFigure(circle, 10, 0) if event == 'LEFT': graph.MoveFigure(circle, -10,0) if event == 'UP': graph.MoveFigure(circle, 0, 10) if event == 'DOWN': graph.MoveFigure(circle, 0,-10) if event=="graph+UP": x2,y2= values['graph'] graph.MoveFigure(circle, x2-x1, y2-y1) x1,y1=x2,y2 window.close()
运行上面的程序。 使用按钮移动圆圈。
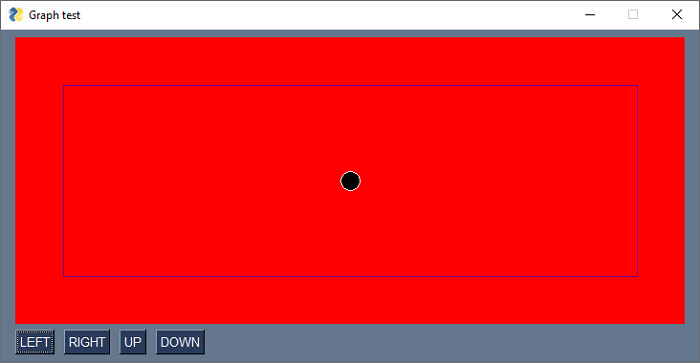