C++ if...else 语句
if 语句后可以跟可选的 else 语句,当布尔表达式为 false 时执行该语句。
语法
C++ 中 if...else 语句的语法为 -
if(boolean_expression) { // 如果布尔表达式为 true,则执行语句 } else { // 如果布尔表达式为 false,则执行语句 }
如果布尔表达式的计算结果为 true,则执行 if 代码块,否则执行 else 代码块。
流程图
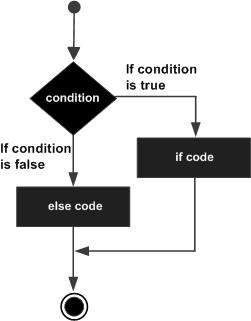
示例
#include <iostream> using namespace std; int main () { // 局部变量声明: int a = 100; // 检查布尔条件 if( a < 20 ) { // 如果条件为真,则打印以下内容 cout << "a is less than 20;" << endl; } else { // 如果条件为假,则打印以下内容 cout << "a is not less than 20;" << endl; } cout << "value of a is : " << a << endl; return 0; }
当编译并执行上述代码时,它会产生以下结果 -
a is not less than 20; value of a is : 100
if...else if...else 语句
一个 if 语句后可以跟一个可选的 else if...else 语句,这对于使用单个 if...else if 语句测试各种条件非常有用。
使用 if、else if、else 语句时,需要注意以下几点。
一个 if 语句可以包含零个或一个 else 语句,并且 else 语句必须位于任何 else if 语句之后。
一个 if 语句可以包含零到多个 else if 语句,并且 else 语句必须位于 else 语句之前。
一旦 else if 语句执行成功,其余的 else if 语句或 else 语句将不再被测试。
语法
C++ 中 if...else if...else 语句的语法为 -
if(boolean_expression 1) { // 当布尔表达式 1 为真时执行 } else if( boolean_expression 2) { // 当布尔表达式 2 为真时执行 } else if( boolean_expression 3) { // 当布尔表达式 3 为真时执行 } else { // 当以上条件都不为真时执行。 }
示例
#include <iostream> using namespace std; int main () { // 局部变量声明: int a = 100; // 检查布尔条件 if( a == 10 ) { // 如果条件为真,则打印以下内容 cout << "Value of a is 10" << endl; } else if( a == 20 ) { // if else if 条件为真 cout << "Value of a is 20" << endl; } else if( a == 30 ) { // if else if 条件为真 cout << "Value of a is 30" << endl; } else { // 如果所有条件都不成立 cout << "Value of a is not matching" << endl; } cout << "Exact value of a is : " << a << endl; return 0; }
当编译并执行上述代码时,它会产生以下结果 -
Value of a is not matching Exact value of a is : 100